|
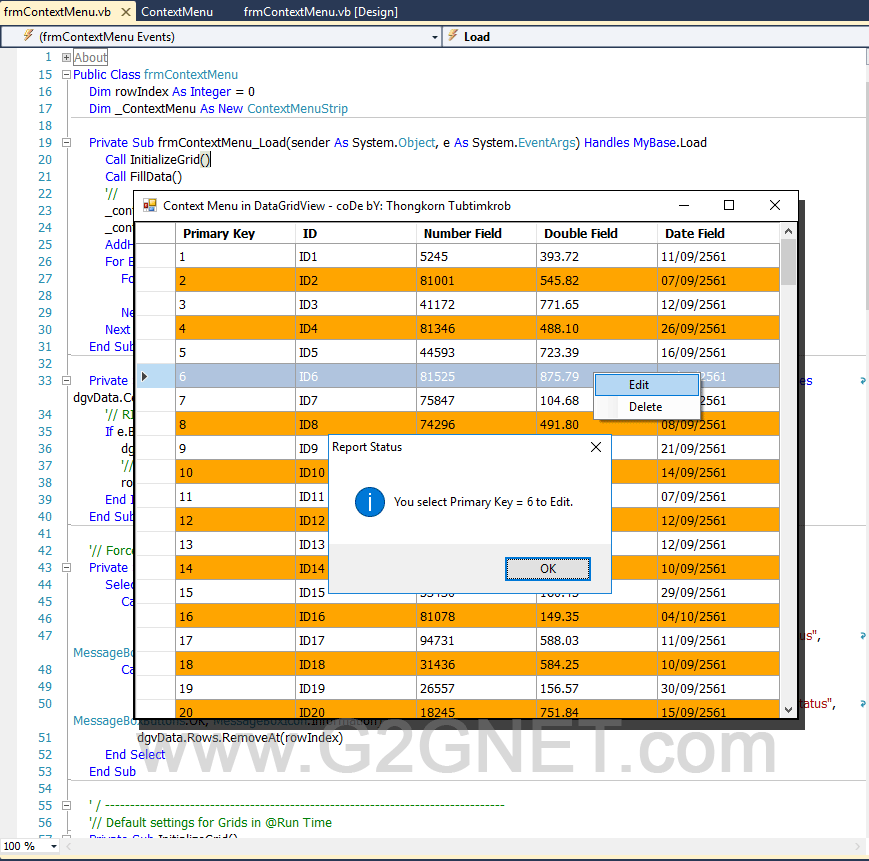
Context Menu คือ หน้าเมนู Pop up ที่แสดงขึ้นเวลาที่เราทำการกดคลิกขวาที่เมาส์ ซึ่งจะแสดงรายละเอียดหรือทางลัดต่างๆตามที่เรากำหนด วันนี้แอดมินจะขอนำเสนอการสร้าง Context Menu เพื่อแสดงผลในตารางกริด (DataGridView) ด้วยวิธีการแบบ @Run Time หมายความว่า เราไม่ได้ทำการลาก ContextMenuStrip มาจากกลุ่มของเครื่องมือ (ToolBox) แต่สร้างขึ้นมาจากโค้ดแทน ซึ่งเป็นวิธีการที่ยืดหยุ่น และทรงประสิทธิภาพในการเขียนโปรแกรมด้วยภาษา Visual Basic ... ดังนั้นมิตรรักแฟนคลับจึงจำเป็นที่ต้องศึกษาโดยผ่านทางโค้ดแทนครับผม โดยตัวอย่างนี้จะเกิดการคลิกขวาที่เมาส์ จากนั้นให้ทำการเลือกแก้ไข (Edit) หรือลบแถวรายการ (Delete) ออกไป ...
การสร้าง Context Menu แบบ Run Time ...
- Dim _ContextMenu As New ContextMenuStrip
- '// Add new contextmenu
- _contextmenu.Items.Add("Edit")
- _contextmenu.Items.Add("Delete")
- AddHandler _ContextMenu.ItemClicked, AddressOf Contextmenu_Click
- '// IMPORTANT
- For Each rw As DataGridViewRow In dgvData.Rows
- For Each c As DataGridViewCell In rw.Cells
- c.ContextMenuStrip = _ContextMenu
- Next
- Next
คัดลอกไปที่คลิปบอร์ด
เหตุการณ์ตรวจสอบการคลิกขวาที่เมาส์หรือไม่ ...
- Private Sub dgvData_CellMouseUp(sender As Object, e As System.Windows.Forms.DataGridViewCellMouseEventArgs) Handles dgvData.CellMouseUp
- '// RIGHT CLICKS
- If e.Button = Windows.Forms.MouseButtons.Right Then
- dgvData.Rows(e.RowIndex).Selected = True
- '// Get the row.
- rowIndex = e.RowIndex
- End If
- End Sub
คัดลอกไปที่คลิปบอร์ด
เหตุการณ์ที่เราสร้างขึ้นมา เพื่อให้เกิด Driven หรือสั่งไปทำอะไร ... ตัวอย่างนี้คือการเลือกรายการแถวมาแก้ไข หรือลบแถวออกไป
- '// Force Event contextmenu_Click
- Private Sub Contextmenu_Click(ByVal sender As System.Object, ByVal e As ToolStripItemClickedEventArgs)
- '// Choose item from contextmenu.
- Select Case e.ClickedItem.Text
- Case "Edit"
- _ContextMenu.Visible = False
- MessageBox.Show("You select Primary Key = " & dgvData.Rows(rowIndex).Cells(0).Value & " to Edit.", "Report Status", MessageBoxButtons.OK, MessageBoxIcon.Information)
- Case "Delete"
- _ContextMenu.Visible = False
- MessageBox.Show("You select Primary Key = " & dgvData.Rows(rowIndex).Cells(0).Value & " to Delete.", "Report Status", MessageBoxButtons.OK, MessageBoxIcon.Information)
- dgvData.Rows.RemoveAt(rowIndex)
- End Select
- End Sub
คัดลอกไปที่คลิปบอร์ด
มาดูโค้ดฉบับเต็มกันเถอะ ...
- #Region "About"
- ' / --------------------------------------------------------------------
- ' / Developer : Mr.Surapon Yodsanga (Thongkorn Tubtimkrob)
- ' / eMail : thongkorn@hotmail.com
- ' / URL: http://www.g2gnet.com (Khon Kaen - Thailand)
- ' / Facebook: https://www.facebook.com/g2gnet (For Thailand)
- ' / Facebook: https://www.facebook.com/commonindy (Worldwide)
- ' / Purpose: Context Menu in DataGridView @Run Time.
- ' / Microsoft Visual Basic .NET (2010)
- ' /
- ' / This is open source code under @CopyLeft by Thongkorn Tubtimkrob.
- ' / You can modify and/or distribute without to inform the developer.
- ' / --------------------------------------------------------------------
- #End Region
- Public Class frmContextMenu
- Dim rowIndex As Integer = 0
- Dim _ContextMenu As New ContextMenuStrip
- Private Sub frmContextMenu_Load(sender As System.Object, e As System.EventArgs) Handles MyBase.Load
- Call InitializeGrid()
- Call FillSampleData()
- '// Add new contextmenu
- _contextmenu.Items.Add("Edit")
- _contextmenu.Items.Add("Delete")
- AddHandler _ContextMenu.ItemClicked, AddressOf Contextmenu_Click
- '// IMPORTANT
- For Each rw As DataGridViewRow In dgvData.Rows
- For Each c As DataGridViewCell In rw.Cells
- c.ContextMenuStrip = _ContextMenu
- Next
- Next
- End Sub
- Private Sub dgvData_CellMouseUp(sender As Object, e As System.Windows.Forms.DataGridViewCellMouseEventArgs) Handles dgvData.CellMouseUp
- '// RIGHT CLICKS
- If e.Button = Windows.Forms.MouseButtons.Right Then
- dgvData.Rows(e.RowIndex).Selected = True
- '// Get the row.
- rowIndex = e.RowIndex
- End If
- End Sub
- '// Force Event contextmenu_Click
- Private Sub Contextmenu_Click(ByVal sender As System.Object, ByVal e As ToolStripItemClickedEventArgs)
- '// Choose item from contextmenu.
- Select Case e.ClickedItem.Text
- Case "Edit"
- _ContextMenu.Visible = False
- MessageBox.Show("You select Primary Key = " & dgvData.Rows(rowIndex).Cells(0).Value & " to Edit.", "Report Status", MessageBoxButtons.OK, MessageBoxIcon.Information)
- Case "Delete"
- _ContextMenu.Visible = False
- MessageBox.Show("You select Primary Key = " & dgvData.Rows(rowIndex).Cells(0).Value & " to Delete.", "Report Status", MessageBoxButtons.OK, MessageBoxIcon.Information)
- dgvData.Rows.RemoveAt(rowIndex)
- End Select
- End Sub
- ' / --------------------------------------------------------------------------------
- '// Default settings for Grids in @Run Time
- Private Sub InitializeGrid()
- With dgvData
- .RowHeadersVisible = False
- .AllowUserToAddRows = False
- .AllowUserToDeleteRows = False
- .AllowUserToResizeRows = False
- .MultiSelect = False
- .SelectionMode = DataGridViewSelectionMode.FullRowSelect
- .ReadOnly = True
- .Font = New Font("Tahoma", 9)
- .RowHeadersVisible = True
- .AlternatingRowsDefaultCellStyle.BackColor = Color.Orange
- .DefaultCellStyle.SelectionBackColor = Color.LightSteelBlue
- '/ Auto size column width of each main by sorting the field.
- .AutoSizeColumnsMode = DataGridViewAutoSizeColumnsMode.Fill
- .AutoResizeColumns()
- '/ Adjust Header Styles
- With .ColumnHeadersDefaultCellStyle
- .BackColor = Color.Navy
- .ForeColor = Color.White
- .Font = New Font("Tahoma", 9, FontStyle.Bold)
- End With
- End With
- End Sub
- ' / --------------------------------------------------------------------------------
- ' / SAMPLE DATA INTO DATAGRIDVIEW
- Private Sub FillSampleData()
- Dim dt As New DataTable
- dt.Columns.Add("Primary Key")
- dt.Columns.Add("ID")
- dt.Columns.Add("Number Field")
- dt.Columns.Add("Double Field")
- dt.Columns.Add("Date Field")
- Dim RandomClass As New Random()
- For i As Long = 0 To 199
- Dim dr As DataRow = dt.NewRow()
- dr(0) = i + 1
- dr(1) = "ID" & i + 1
- dr(2) = RandomClass.Next(1, 99999)
- dr(3) = FormatNumber(RandomClass.Next(100, 1000) + RandomClass.NextDouble(), 2)
- '// Random Date
- Dim d As Date = Date.Today
- d = d.AddDays(RandomClass.Next(-30, 0))
- dr(4) = FormatDateTime(d, DateFormat.ShortDate).ToString
- dt.Rows.Add(dr)
- Next
- dgvData.DataSource = dt
- End Sub
- Private Sub frmContextMenu_FormClosed(sender As Object, e As System.Windows.Forms.FormClosedEventArgs) Handles Me.FormClosed
- Me.Dispose()
- Application.Exit()
- End Sub
- End Class
คัดลอกไปที่คลิปบอร์ด
ดาวน์โหลดโค้ดฉบับเต็ม VB.NET (2010) ได้ที่นี่ ...
|
ขออภัย! โพสต์นี้มีไฟล์แนบหรือรูปภาพที่ไม่ได้รับอนุญาตให้คุณเข้าถึง
คุณจำเป็นต้อง ลงชื่อเข้าใช้ เพื่อดาวน์โหลดหรือดูไฟล์แนบนี้ คุณยังไม่มีบัญชีใช่ไหม? ลงทะเบียน
x
|