|
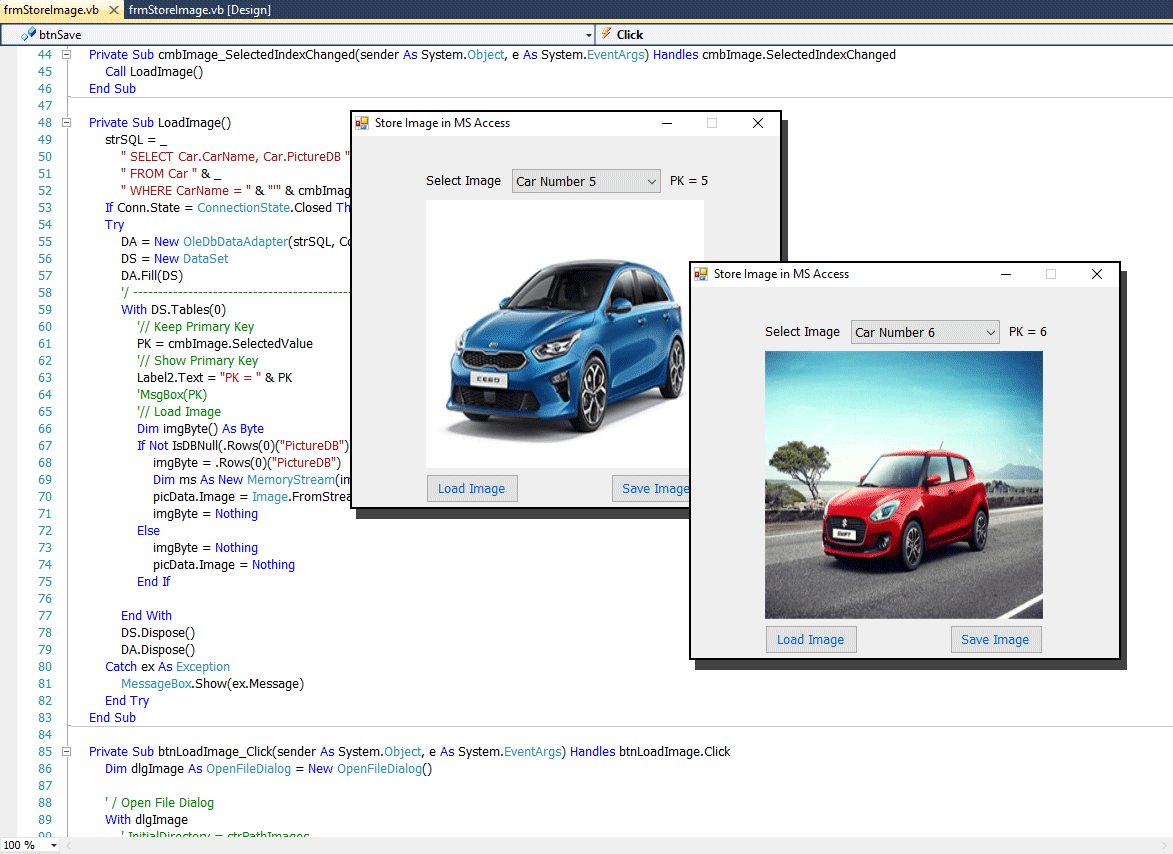
ชนิดข้อมูลแบบ Binary Large OBject (BLOB) หรือวัตถุขนาดใหญ่แบบไบนารี ชนิดข้อมูลนี้เหมาะสำหรับเก็บรูปภาพ, เสียง, กราฟิก หากถามแอดมินว่าเหมาะสมหรือไม่ในการจัดเก็บเข้าสู่ฐานข้อมูล ตอบว่าไม่ดีเลยครับ เพราะจะทำให้ฐานข้อมูลมีขนาดใหญ่โตเทอะทะเอาซ่ะเปล่าๆ แต่ที่แอดมินเอาโค้ดมานำเสนอให้ดู ก็เพื่อให้ไว้ศึกษา หรือประยุกต์ใช้กับงานขนาดเล็กๆก็แล้วกันครับผม โค้ดนี้จะแสดงการโหลดรูปภาพออกมาจากตารางข้อมูล แล้วให้เลือก Browse ไฟล์ภาพใหม่ จากนั้นทำการบันทึกเข้าไปเก็บลงในฐานข้อมูล ส่วนการเพิ่มข้อมูล (Add) แอดมินอยากให้ไปคิดเพิ่มเติมกันเอาเองน่ะข่ะรับกระผม
มาดูโค้ดฉบับเต็มกันเถอะ ...
- ' / --------------------------------------------------------------------------------
- ' / Developer : Mr.Surapon Yodsanga (Thongkorn Tubtimkrob)
- ' / eMail : thongkorn@hotmail.com
- ' / URL: http://www.g2gnet.com (Khon Kaen - Thailand)
- ' / Facebook: https://www.facebook.com/g2gnet (For Thailand)
- ' / Facebook: https://www.facebook.com/commonindy (Worldwide)
- ' / More Info: http://www.g2gnet.com/webboard
- ' /
- ' / Purpose: Load and save images with BLOB into MS Access.
- ' / Microsoft Visual Basic .NET (2010) + MS Access 2007+
- ' /
- ' / This is open source code under @CopyLeft by Thongkorn Tubtimkrob.
- ' / You can modify and/or distribute without to inform the developer.
- ' / --------------------------------------------------------------------------------
- Imports System.Data.OleDb
- Imports System.IO
- Public Class frmStoreImage
- '// Declare public variables only this form.
- Dim Conn As OleDbConnection
- Dim Cmd As OleDbCommand
- Dim DS As DataSet
- Dim DR As OleDbDataReader
- Dim DA As OleDbDataAdapter
- Dim strSQL As String '// Major SQL
- '// Define Path
- Dim strPathData As String = MyPath(Application.StartupPath)
- '// Primary Key
- Dim PK As Long
- Private Sub frmStoreImage_Load(sender As System.Object, e As System.EventArgs) Handles MyBase.Load
- Call ConnectDataBase()
- Call LoadComboBox()
- End Sub
- ' / Load table detail into ComboBox
- Public Sub LoadComboBox()
- If Conn.State = ConnectionState.Closed Then Conn.Open()
- Try
- strSQL = "SELECT * FROM Car ORDER BY CarID "
- Cmd = New OleDb.OleDbCommand(strSQL, Conn)
- DR = Cmd.ExecuteReader
- Dim DT As DataTable = New DataTable
- DT.Load(DR)
- '/ Primary Key (ValueMember)
- cmbImage.ValueMember = "CarPK"
- '/ Display the name
- cmbImage.DisplayMember = "CarName"
- cmbImage.DataSource = DT
- DR.Close()
- Cmd.Dispose()
- Conn.Close()
- Catch ex As Exception
- MessageBox.Show(ex.Message)
- End Try
- End Sub
- Private Sub cmbImage_SelectedIndexChanged(sender As System.Object, e As System.EventArgs) Handles cmbImage.SelectedIndexChanged
- Call LoadImage()
- End Sub
- Private Sub LoadImage()
- strSQL = _
- " SELECT Car.CarName, Car.PictureDB " & _
- " FROM Car " & _
- " WHERE CarName = " & "'" & cmbImage.Text & "'"
- If Conn.State = ConnectionState.Closed Then Conn.Open()
- Try
- DA = New OleDbDataAdapter(strSQL, Conn)
- DS = New DataSet
- DA.Fill(DS)
- '/ ------------------------------------------------------------------
- With DS.Tables(0)
- '// Keep Primary Key
- PK = cmbImage.SelectedValue
- '// Show Primary Key
- Label2.Text = "PK = " & PK
- 'MsgBox(PK)
- '// Load Image
- Dim imgByte() As Byte
- If Not IsDBNull(.Rows(0)("PictureDB")) Then
- imgByte = .Rows(0)("PictureDB")
- Dim ms As New MemoryStream(imgByte)
- picData.Image = Image.FromStream(ms)
- imgByte = Nothing
- Else
- imgByte = Nothing
- picData.Image = Nothing
- End If
- End With
- DS.Dispose()
- DA.Dispose()
- Catch ex As Exception
- MessageBox.Show(ex.Message)
- End Try
- End Sub
- Private Sub btnLoadImage_Click(sender As System.Object, e As System.EventArgs) Handles btnLoadImage.Click
- Dim dlgImage As OpenFileDialog = New OpenFileDialog()
- ' / Open File Dialog
- With dlgImage
- '.InitialDirectory = strPathImages
- .Title = "Select images"
- .Filter = "Images types (*.jpg;*.png;*.gif;*.bmp)|*.jpg;*.png;*.gif;*.bmp"
- .FilterIndex = 1
- .RestoreDirectory = True
- End With
- ' Select OK after Browse ...
- If dlgImage.ShowDialog() = DialogResult.OK Then
- '// New Image
- picData.Image = Image.FromFile(dlgImage.FileName)
- End If
- End Sub
- ' / --------------------------------------------------------------------------------
- ' / Save Images with Primary Key from SelectValue ComboBox.
- Private Sub btnSave_Click(sender As System.Object, e As System.EventArgs) Handles btnSave.Click
- strSQL = _
- " UPDATE Car SET " & _
- " CarPK= @CPK, PictureDB = @PICDB " & _
- " WHERE CarPK= @CPK "
- If Conn.State = ConnectionState.Closed Then Conn.Open()
- Cmd = New OleDb.OleDbCommand
- '// Images from PictureBox
- Dim imgByte As Byte()
- Dim MemStream As New MemoryStream
- '// Have to load image into PictureBox or not?
- If Not IsNothing(Me.picData.Image) Then
- Me.picData.Image.Save(MemStream, Imaging.ImageFormat.Jpeg)
- imgByte = MemStream.GetBuffer()
- MemStream.Read(imgByte, 0, MemStream.Length)
- Else
- imgByte = Nothing
- End If
- '// START
- Try
- Cmd.Parameters.AddWithValue("@CPK", PK)
- Cmd.Parameters.AddWithValue("@PICDB", IIf(imgByte IsNot Nothing, imgByte, DBNull.Value))
- '//
- Cmd.Connection = Conn
- Cmd.CommandType = CommandType.Text
- Cmd.CommandText = strSQL
- Cmd.ExecuteNonQuery()
- imgByte = Nothing
- MemStream.Close()
- '// Processing ...
- MessageBox.Show("Records Updated Completed.", "Update Status", MessageBoxButtons.OK, MessageBoxIcon.Information)
- Catch ex As Exception
- MessageBox.Show(ex.Message)
- End Try
- Cmd.Parameters.Clear()
- Conn.Close()
- End Sub
- ' / --------------------------------------------------------------------------------
- Public Sub ConnectDataBase()
- Dim strConn As String = _
- "Provider = Microsoft.ACE.OLEDB.12.0;"
- strConn += _
- "Data Source = " & strPathData & "SampleDB.accdb"
- Try
- '/ Create a connection
- Conn = New OleDbConnection(strConn)
- '/ Open the connection and execute the select command
- Conn.Open()
- Catch ex As Exception
- MessageBox.Show(ex.Message)
- End Try
- End Sub
- ' / --------------------------------------------------------------------------------
- ' / Get my project path
- ' / AppPath = C:\My Project\bin\debug
- ' / Replace "\bin\debug" with ""
- ' / Return : C:\My Project\
- Function MyPath(AppPath As String) As String
- '/ MessageBox.Show(AppPath);
- AppPath = AppPath.ToLower()
- '/ Return Value
- MyPath = AppPath.Replace("\bin\debug", "").Replace("\bin\release", "").Replace("\bin\x86\debug", "")
- '// If not found folder then put the \ (BackSlash ASCII Code = 92) at the end.
- If Microsoft.VisualBasic.Right(MyPath, 1) <> Chr(92) Then MyPath = MyPath & Chr(92)
- End Function
- Private Sub frmStoreImage_FormClosed(sender As Object, e As System.Windows.Forms.FormClosedEventArgs) Handles Me.FormClosed
- Me.Dispose()
- Application.Exit()
- End Sub
- End Class
คัดลอกไปที่คลิปบอร์ด
ดาวน์โหลดโค้ดต้นฉบับ VB.NET (2010) ได้ที่นี่ ...
|
ขออภัย! โพสต์นี้มีไฟล์แนบหรือรูปภาพที่ไม่ได้รับอนุญาตให้คุณเข้าถึง
คุณจำเป็นต้อง ลงชื่อเข้าใช้ เพื่อดาวน์โหลดหรือดูไฟล์แนบนี้ คุณยังไม่มีบัญชีใช่ไหม? ลงทะเบียน
x
|