|
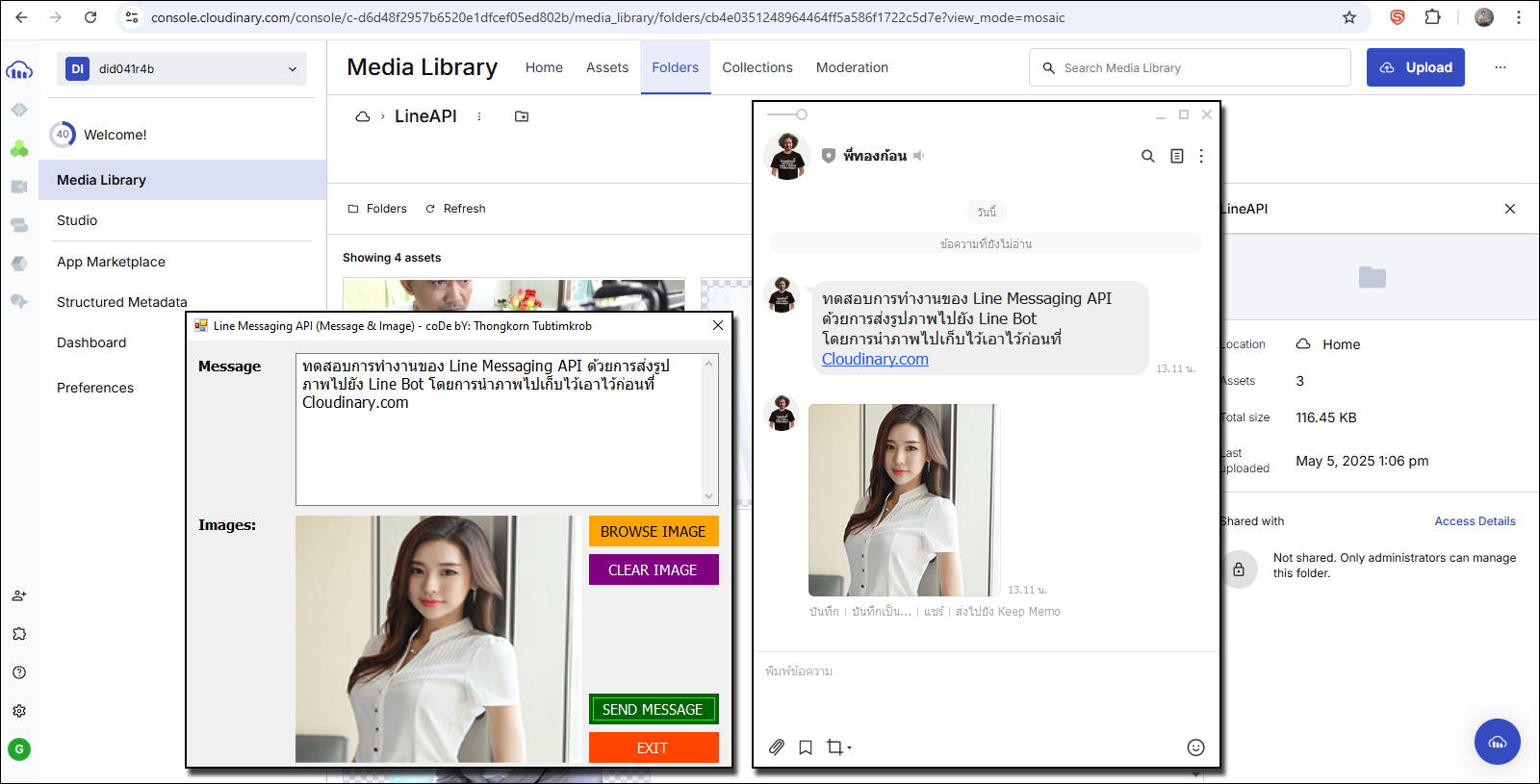
*** จะต้องติดตั้ง CloudinaryDotNet เข้าไปใหม่ก่อนด้วย เพราะโค้ดต้นฉบับไม่ได้แนบไฟล์ DLL ไปด้วย ***
*** การติดตั้ง CloudinaryDotNet จะติดตั้ง NewTonSoft มาให้อัตโนมัติ ***
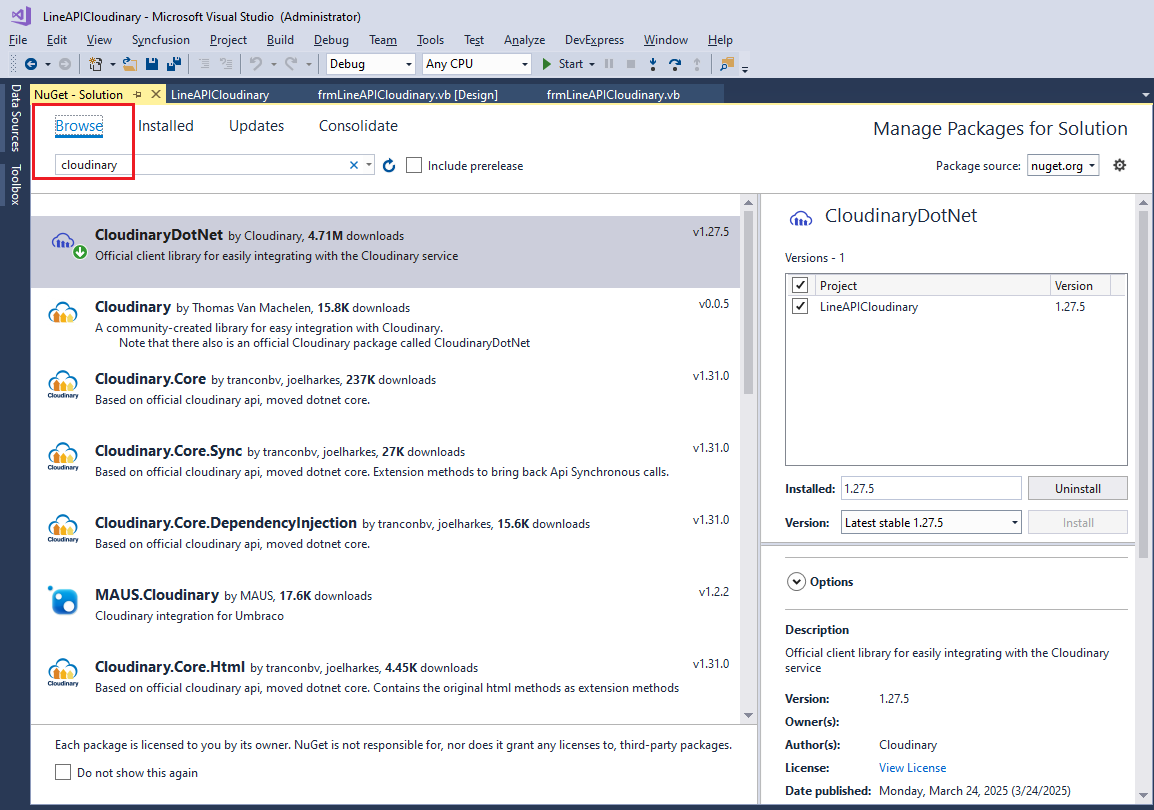
การใช้ Nuget เพื่อทำการติดตั้ง CloudinaryDotNet ... (เป็นที่เก็บไฟล์ Media ต่างๆ)
สมัครใช้งาน Cloudinary.com ได้ที่นี่ ...
คลิปวิดีโอในการสมัครใช้งาน Line Official Account เพื่อรับค่า Channel Access Token และ UserID (สำคัญ)
คลิปวิดีโอประกอบการอธิบายในโค้ดชุดนี้ ...
มาดูโค้ดฉบับเต็มกันเถอะ ... อย่าลืมใส่ค่าต่างๆก่อนที่จะรันโปรแกรมด้วย ...- '// สมัครการใช้งาน Line Messaging API ที่
- '// https://manager.line.biz/
- '// https://developers.line.biz/en/
- '// จากนั้น สร้าง Channel ใน Line Messaging API
- '// รับ Channel Access Token และ User ID จากหน้า Line Console
- '// ติดตั้ง Library Newtonsoft.Json เพื่อช่วยจัดการ JSON
- '// ใน NuGet Package Manager Console ใช้คำสั่ง:
- '// Install-Package Newtonsoft.Json
- '// .Net Framework 4.5
- '// .Net SDK & Cloudinary Document
- '// https://cloudinary.com/documentation/dotnet_integration
- '// Sign Up
- '// https://www.cloudinary.com
- Imports System.IO
- Imports CloudinaryDotNet
- Imports CloudinaryDotNet.Actions
- Imports System.Net.Http
- Imports System.Net.Http.Headers
- Imports Newtonsoft.Json
- Imports System.Text
- Public Class frmLineAPICloudinary
- '// ------------------------------------------------------------------------------------------------
- '// เก็บตำแหน่งและชื่อไฟล์ภาพต้นฉบับที่ต้องการอัพโหลด
- Dim PictureUploadPath As String = String.Empty
- '// แยกชื่อไฟล์+นามสกุล ออกจากโฟลเดอร์
- Dim UploadFileName As String = ""
- '// Default path.
- Dim PictureDefaultPath As String = MyPath(Application.StartupPath) & "Images"
- '// Cloudinary Configuration
- Private Const CLOUD_NAME As String = "CLOUD_NAME"
- Private Const API_KEY As String = "API_KEY"
- Private Const API_SECRET As String = "API_SECRET"
- '// กำหนดโฟลเดอร์ที่เก็บไฟล์บน Cloudinary
- Private Const CLOUD_FOLDER As String = "LineAPI" '// สามารถเปลี่ยนชื่อโฟลเดอร์ได้ใหม่เลย
- '// Line Bot Config
- Private Const LINE_ACCESS_TOKEN As String = ""
- Private Const USER_ID As String = ""
- '// ------------------------------------------------------------------------------------------------
- Private Sub frmLineAPICloudinary_Load(sender As Object, e As EventArgs) Handles MyBase.Load
- txtMessage.Text = "ทดสอบการทำงานของ Line Messaging API ด้วยการส่งข้อความและรูปภาพ"
- End Sub
- '// ------------------------------------------------------------------------------------------------
- '// ส่งข้อความและรูปภาพไปยัง LINE
- '// ------------------------------------------------------------------------------------------------
- Private Sub btnSendMessage_Click(sender As Object, e As EventArgs) Handles btnSendMessage.Click
- '// ตรวจสอบว่าทั้งข้อความและภาพว่างหรือไม่
- If String.IsNullOrWhiteSpace(txtMessage.Text.Trim) AndAlso String.IsNullOrWhiteSpace(PictureUploadPath) Then
- MessageBox.Show("กรุณาใส่ข้อความหรือเลือกภาพก่อนส่ง", "คำเตือน", MessageBoxButtons.OK, MessageBoxIcon.Warning)
- Return
- Else
- '// ทำการส่งข้อความและไฟล์ภาพไปใน Line Bot
- Call UploadImageAndSendToLine(PictureUploadPath, txtMessage.Text)
- End If
- '// Clear
- UploadFileName = ""
- PictureUploadPath = String.Empty
- picData.Image = Image.FromFile(PictureDefaultPath & "NoImage.gif")
- End Sub
- '// ------------------------------------------------------------------------------------------------
- '// คำสั่ง Async ใช้เพื่อระบุว่าฟังก์ชันหรือเมธอดสามารถดำเนินการแบบ Asynchronous
- '// หรือเป็นทำงานแบบไม่รอให้เสร็จสมบูรณ์ก่อนที่จะไปทำคำสั่งถัดไปได้
- '// โดยปกติจะใช้ร่วมกับคำสั่ง Await เพื่อเรียกใช้งานที่ใช้เวลานาน
- '// เช่น การร้องขอข้อมูลจาก API, อ่าน/เขียนไฟล์, หรือรอเครือข่าย โดยไม่บล็อกส่วนที่เหลือของแอพพลิเคชัน
- '// ------------------------------------------------------------------------------------------------
- Public Async Function UploadImageAndSendToLine(ByVal imagePath As String, ByVal message As String) As Task
- Dim account = New Account(CLOUD_NAME, API_KEY, API_SECRET)
- Dim cloudinary = New Cloudinary(account)
- Dim uploadResult As ImageUploadResult = Nothing
- Dim publicId As String = Nothing
- Dim imageUrl As String = String.Empty
- Try
- '// 1. Upload Image to Cloudinary
- If Not String.IsNullOrEmpty(imagePath) AndAlso IO.File.Exists(imagePath) Then
- Dim uploadParams = New ImageUploadParams() With {
- .File = New FileDescription(imagePath),
- .Folder = CLOUD_FOLDER '// Line API folder on Cloudinary.
- }
- uploadResult = cloudinary.Upload(uploadParams)
- imageUrl = uploadResult.SecureUrl.ToString()
- publicId = uploadResult.PublicId
- End If
- '// 2. Send to LINE Messaging API
- Using client As New HttpClient()
- client.DefaultRequestHeaders.Authorization = New AuthenticationHeaderValue("Bearer", LINE_ACCESS_TOKEN)
- '// เตรียมรายการข้อความ สร้าง Payload ตามรูปแบบที่ Line API ต้องการ
- '// Payload คือเนื้อหาข้อมูลที่ส่งไปยัง Line Server ผ่าน API เช่น การส่งข้อความ, การส่งรูปภาพ หรือการสร้าง Flex Message
- '// ประกาศตัวแปร messages เป็นแบบ List(Of Object) จากนั้นค่อยนำไปแปลงให้อยู่ในรูปแบบ JSON Format
- Dim messages = New List(Of Object)
- '// ตรวจสอบว่ามีข้อความ
- If Not String.IsNullOrEmpty(message) Then
- messages.Add(New With {.type = "text", .text = message})
- End If
- '// ตรวจสอบว่ามี URL รูปภาพ
- If Not String.IsNullOrEmpty(imageUrl) Then
- messages.Add(New With {
- .type = "image",
- .originalContentUrl = imageUrl,
- .previewImageUrl = imageUrl
- })
- End If
- Dim payload = New With {
- .to = USER_ID,
- .messages = messages.ToArray()
- }
- '// สำหรับวิดีโอ
- 'If Not String.IsNullOrEmpty(videoUrl) AndAlso Not String.IsNullOrEmpty(videoPreviewUrl) Then
- 'messages.Add(New With {
- 'Key .type = "video",
- 'Key .originalContentUrl = videoUrl,
- 'Key .previewImageUrl = videoPreviewUrl
- '})
- 'End If
- '// แปลงเป็น JSON แล้วส่ง
- Dim json = JsonConvert.SerializeObject(payload, Formatting.Indented)
- '// ตัวอย่าง:
- '// {
- '// "to": "USER_ID",
- '// "messages": [
- '// {
- '// "type": "text",
- '// "text": "สวัสดีครับ! นี่คือข้อความจาก Line Messaging API"
- '// },
- '// {
- '// "type": "image",
- '// "originalContentUrl": "https://example.com/image.jpg",
- '// "previewImageUrl": "https://example.com/image_preview.jpg" (หรือใช้ชื่อไฟล์ภาพ Content ตัวเดียวกันได้เลย)
- '// }
- '// ]
- '// }
- Dim content = New StringContent(json, Encoding.UTF8, "application/json")
- Dim response = Await client.PostAsync("https://api.line.me/v2/bot/message/push", content)
- Dim result = Await response.Content.ReadAsStringAsync()
- If Not response.IsSuccessStatusCode Then
- MessageBox.Show("เกิดข้อผิดพลาด: " & response.StatusCode & vbCrLf & result, "Report Error", MessageBoxButtons.OK, MessageBoxIcon.Error)
- End If
- '// หากต้องการแสดงข้อความแจ้งเตือน
- 'If response.IsSuccessStatusCode Then
- 'MessageBox.Show("ส่งข้อความและรูปภาพสำเร็จ!", "Complete", MessageBoxButtons.OK, MessageBoxIcon.Information)
- 'Else
- 'MessageBox.Show("เกิดข้อผิดพลาด: " & response.StatusCode & vbCrLf & responseBody, "Report Error", MessageBoxButtons.OK, MessageBoxIcon.Error)
- 'End If
- End Using
- Catch ex As Exception
- MessageBox.Show("Error: " & ex.Message)
- '// 3: Delete Image From Cloudinary.
- Finally
- If Not String.IsNullOrEmpty(publicId) Then
- Try
- Dim deletionParams As New DeletionParams(publicId)
- Dim deletionResult = Cloudinary.Destroy(deletionParams)
- 'If deletionResult.Result = "ok" Then
- ' MessageBox.Show("ลบภาพจาก Cloudinary สำเร็จ", "Report Status", MessageBoxButtons.OK, MessageBoxIcon.Information)
- 'Else
- ' MessageBox.Show("ลบภาพไม่สำเร็จ: " & deletionResult.Result, "Report Error", MessageBoxButtons.OK, MessageBoxIcon.Error)
- 'End If
- Catch ex1 As Exception
- MessageBox.Show("ลบภาพไม่สำเร็จ: " & ex1.Message, "Report Error", MessageBoxButtons.OK, MessageBoxIcon.Error)
- End Try
- End If
- End Try
- End Function
- '// ------------------------------------------------------------------------------------------------
- '// เบราซ์หาไฟล์ภาพ
- '// ------------------------------------------------------------------------------------------------
- Private Sub btnBrowse_Click(sender As Object, e As EventArgs) Handles btnBrowse.Click
- Dim dlgImage As OpenFileDialog = New OpenFileDialog()
- '// Open File Dialog
- With dlgImage
- .InitialDirectory = PictureDefaultPath
- .Title = "Select Image File"
- .Filter = "Format (*.jpg;*.jpeg;*.png)|*.jpg;*.jpeg;*.png"
- .FilterIndex = 1
- .RestoreDirectory = True
- End With
- '// Select OK after Browse ...
- If dlgImage.ShowDialog() = DialogResult.OK Then
- '// ตรวจสอบก่อนว่าขนาดไฟล์เกิน 1 MB. หรือไม่ (Cloudinary น่าจะอัพโหลดได้สูงสุด 10 MB.)
- Dim info As New FileInfo(dlgImage.FileName)
- If (info.Length / 1024) > 1024 Then
- MessageBox.Show("The image file you selected is of size " & Format((info.Length / 1024), "#,##0") & " KB. is too big more than 1,024 KB.", "Report Status", MessageBoxButtons.OK, MessageBoxIcon.Warning)
- Exit Sub
- End If
- '// Put the current image file into PictureBox Control.
- picData.Image = Image.FromFile(dlgImage.FileName)
- '// เอาเฉพาะชื่อไฟล์และนามสกุลภาพ (Filename + Extension) เช่น thongkorn.png
- UploadFileName = dlgImage.SafeFileName
- '// เอาไฟล์ภาพมาเต็มพาธ เพื่อระบุต้นทางไฟล์ก่อนทำการอัพโหลด
- PictureUploadPath = dlgImage.FileName
- End If
- End Sub
- '// ------------------------------------------------------------------------------------------------
- Private Sub btnClear_Click(sender As Object, e As EventArgs) Handles btnClear.Click
- picData.Image = Image.FromFile(PictureDefaultPath & "NoImage.gif")
- UploadFileName = ""
- PictureUploadPath = String.Empty
- End Sub
- Private Sub btnExit_Click(sender As Object, e As EventArgs) Handles btnExit.Click
- Me.Close()
- End Sub
- Private Sub frmLineAPICloudinary_FormClosed(sender As Object, e As FormClosedEventArgs) Handles Me.FormClosed
- Me.Dispose()
- GC.SuppressFinalize(Me)
- Application.Exit()
- End Sub
- #Region "FUNCTION"
- '// / --------------------------------------------------------------------------------
- '// / Get my project path
- '// / AppPath = C:\My Project\bin\debug
- '// / Replace "\bin\debug" with ""
- '// / Return : C:\My Project\
- Function MyPath(ByVal AppPath As String) As String
- '/ MessageBox.Show(AppPath);
- AppPath = AppPath.ToLower()
- '/ Return Value
- MyPath = AppPath.Replace("\bin\debug", "").Replace("\bin\release", "").Replace("\bin\x86\debug", "")
- '// If not found folder then put the \ (BackSlash) at the end.
- If Microsoft.VisualBasic.Right(MyPath, 1) <> Chr(92) Then MyPath = MyPath & Chr(92)
- End Function
- #End Region
- End Class
คัดลอกไปที่คลิปบอร์ด
ดาวน์โหลดโค้ดต้นฉบับ VB.NET & .Net Framework 4.5.2+ ได้ที่นี่ ...
|
ขออภัย! โพสต์นี้มีไฟล์แนบหรือรูปภาพที่ไม่ได้รับอนุญาตให้คุณเข้าถึง
คุณจำเป็นต้อง ลงชื่อเข้าใช้ เพื่อดาวน์โหลดหรือดูไฟล์แนบนี้ คุณยังไม่มีบัญชีใช่ไหม? ลงทะเบียน
x
|