|
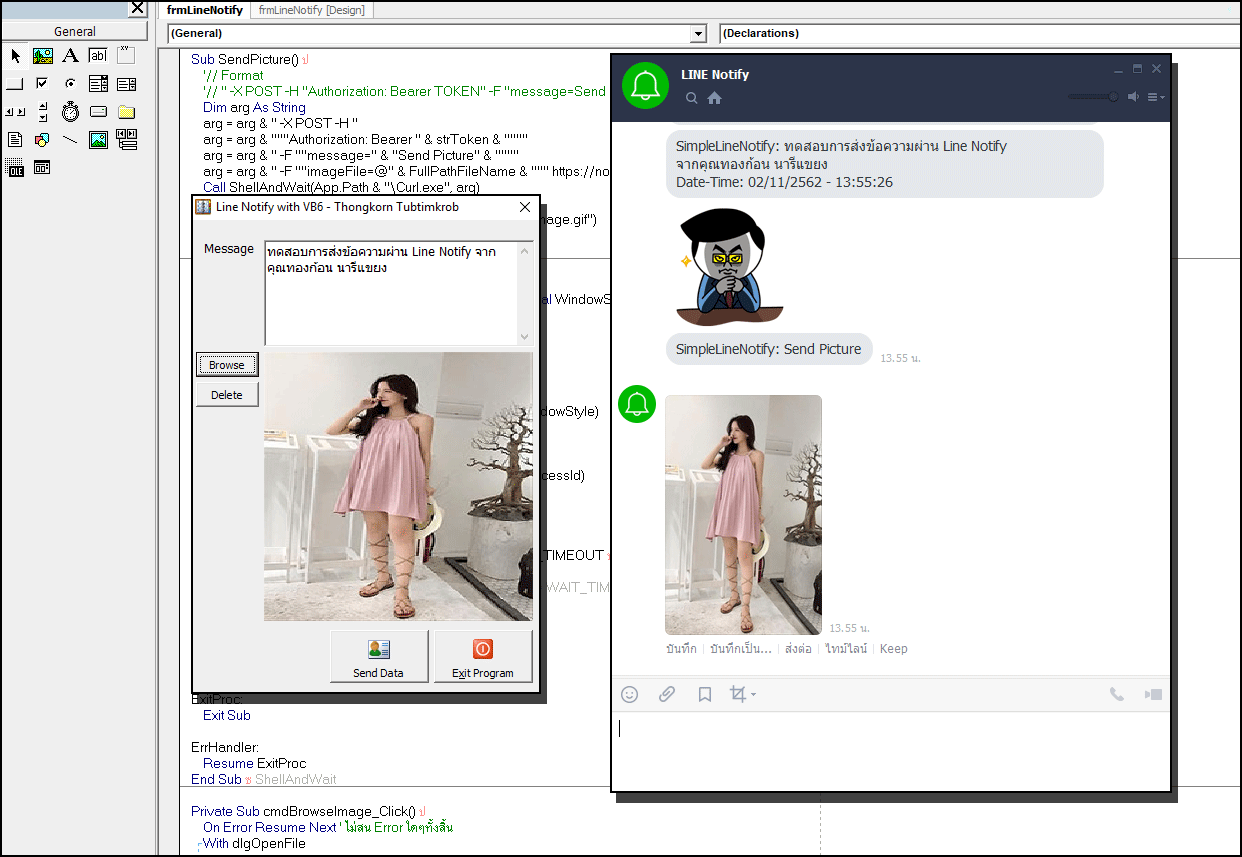
จากบทความ [VB.NET] การทำ Line Notify และอัพโหลดรูปภาพจากตัวโปรแกรม ด้วยการใช้คำสั่ง cURL จากการใช้งานคำสั่งแบบ Command Line ด้วย cURL ทำให้แอดมินได้ค้นพบความรู้ใหม่ในการใช้ Windows Application จาก Visual Basic เพื่อประสานการทำงานร่วมกันกับบรรดา API ที่ใช้งานอยู่บนไดนามิคเว็บเพจ แต่สำหรับ VB6 มันไม่มีคำสั่งสำเร็จรูป หรือ Build In มาให้เหมือนกับ VB.NET ดังนั้นเราจึงต้องเรียกใช้ความสามารถพิเศษเพิ่มเติมจากในตัว Windows หรือที่เรียกว่า WinAPI32 มาใช้งานกับโปรเจคนี้ โดยการสร้างโปรแกรมย่อย ShellAndWait คือการเรียก Shell เพื่อแยกการประมวลผลออกมา แล้วรอคอยจนกว่ามันจะทำงานเสร็จสมบูรณ์เรียบร้อย ...
ดาวน์โหลด cURL สำหรับ Windows ทั้ง 32 บิตและ 64 บิต ... เมื่อดาวน์โหลดเรียบร้อยให้แตกไฟล์ออกมา แล้วไปที่โฟลเดอร์ Bin จะมีอยู่ 3 ไฟล์ และต้องนำไฟล์ทั้ง 3 ตัวของ cURL ไปเก็บไว้ในโฟลเดอร์ที่เราสั่งรันโปรแกรม หรือตำแหน่งที่เรากำหนดเองก็ได้
รูปแบบของการ POST ไปยัง Line ...
- '// Format
- '// " -X POST -H "Authorization: Bearer TOKEN" -F "message=Send Picture" -F "imageFile=@D:\Sample.jpg" https://notify-api.line.me/api/notify"
คัดลอกไปที่คลิปบอร์ด
มาดูโค้ดฉบับเต็มกันเถอะ ...
- ' / --------------------------------------------------------------------------------
- ' / Developer : Mr.Surapon Yodsanga (Thongkorn Tubtimkrob)
- ' / eMail : thongkorn@hotmail.com
- ' / URL: http://www.g2gnet.com (Khon Kaen - Thailand)
- ' / Facebook: https://www.facebook.com/g2gnet (For Thailand)
- ' / Facebook: https://www.facebook.com/commonindy (Worldwide)
- ' / Purpose: Line Notify and Upload image with Visual Basic 6.
- ' / Microsoft Visual Basic 6.0 (SP6)
- ' /
- ' / This is open source code under @CopyLeft by Thongkorn Tubtimkrob.
- ' / You can modify and/or distribute without to inform the developer.
- ' / --------------------------------------------------------------------------------
- Option Explicit
- Private Declare Function OpenProcess Lib "kernel32" ( _
- ByVal dwDesiredAccess As Long, _
- ByVal bInheritHandle As Long, _
- ByVal dwProcessId As Long _
- ) As Long
- Private Declare Function WaitForSingleObject Lib "kernel32" ( _
- ByVal hHandle As Long, _
- ByVal dwMilliseconds As Long _
- ) As Long
- Private Declare Function CloseHandle Lib "kernel32" (ByVal hObject As Long) As Long
- Const WAIT_TIMEOUT = &H102
- Const SYNCHRONIZE As Long = &H100000
- Const INFINITE As Long = &HFFFFFFFF
- Dim FullPathFileName As String
- '// Line Notify Access Token
- Const strToken As String = "YOUR TOKEN"
- Private Sub Form_Load()
- txtMessage.Text = "ทดสอบการส่งข้อความผ่าน Line Notify จากคุณทองก้อน นารีแขยง"
- Image1.Picture = LoadPicture(App.Path & "\Images\NoImage.gif")
- FullPathFileName = ""
- End Sub
- Private Sub cmdSend_Click()
- If Trim$(txtMessage.Text) = "" Or Len(Trim$(txtMessage.Text)) = 0 Then Exit Sub
- Call SendMessage
- If FullPathFileName <> "" Or Len(FullPathFileName) <> 0 Then Call SendPicture
- End Sub
- Sub SendMessage()
- On Error GoTo ErrHandler
-
- Dim oXML As Object
-
- Dim strMessage As String
- Dim strDate As String
- Dim URL As String
- '// Line Notify
- URL = "https://notify-api.line.me/api/notify"
- strMessage = Trim(txtMessage.Text)
- strDate = Format(Now, "dd/MM/yyyy - hh:mm:ss")
- '//Line Message
- strMessage = "message=" & strMessage & vbCrLf & "Date-Time: " & strDate
- 'strMessage = strMessage & "&stickerPackageId=1" & "&stickerId=109"
- '//
- Set oXML = CreateObject("Microsoft.XMLHTTP")
- With oXML
- '// Line POST Method
- .Open "POST", URL, 0
- '// Header
- .SetRequestHeader "Content-Type", "application/x-www-form-urlencoded"
- .SetRequestHeader "Authorization", "Bearer " & strToken
- '// Send message
- .send (strMessage)
- '// Debug Line
- Debug.Print oXML.responseText
- End With
-
- Set oXML = Nothing
- Exit Sub
-
- ErrHandler:
- '// Error
- MsgBox Err.Number & vbCrLf & Err.Description
- End Sub
- Sub SendPicture()
- '// Format
- '// " -X POST -H "Authorization: Bearer TOKEN" -F "message=Send Picture" -F "imageFile=@D:\Sample.jpg" https://notify-api.line.me/api/notify"
- Dim arg As String
- arg = arg & " -X POST -H "
- arg = arg & """Authorization: Bearer " & strToken & """"
- arg = arg & " -F ""message=" & "Send Picture" & """"
- arg = arg & " -F ""imageFile=@" & FullPathFileName & """ https://notify-api.line.me/api/notify"
- Call ShellAndWait(App.Path & "\Curl.exe", arg)
- '// Clear Image
- Image1.Picture = LoadPicture(App.Path & "\Images\NoImage.gif")
- FullPathFileName = ""
- End Sub
- '// Process command line.
- Public Sub ShellAndWait(PathName, args As String, Optional WindowStyle As VbAppWinStyle = vbMinimizedFocus, Optional b As Boolean = False)
-
- On Error GoTo ErrHandler
-
- Dim dwProcessId As Long
- Dim hProcess As Long
- '// Process Shell
- dwProcessId = Shell(PathName & Space$(1) & args, WindowStyle)
-
- If dwProcessId = 0 Then Exit Sub
-
- hProcess = OpenProcess(SYNCHRONIZE, False, dwProcessId)
-
- If hProcess = 0 Then Exit Sub
-
- If b Then
- Do While WaitForSingleObject(hProcess, 100) = WAIT_TIMEOUT
- DoEvents
- Loop
- Else
- WaitForSingleObject hProcess, INFINITE
- End If
-
- CloseHandle hProcess
-
- ExitProc:
- Exit Sub
-
- ErrHandler:
- Resume ExitProc
- End Sub
- Private Sub cmdBrowseImage_Click()
- On Error Resume Next
- With dlgOpenFile
- .FileName = ""
- .InitDir = App.Path & "\Images"
- .DialogTitle = " เลือกไฟล์ภาพ - Graphics File Format "
- .Filter = "ไฟล์ภาพ (*.jpg;*.gif;*.bmp)|*.jpg;*.gif;*.bmp"
- .CancelError = True
- .ShowOpen
- End With
-
- FullPathFileName = dlgOpenFile.FileName
-
- If Trim(FullPathFileName) = "" Or Len(FullPathFileName) = 0 Then Exit Sub
-
- Image1.Picture = LoadPicture(FullPathFileName)
- End Sub
- Private Sub cmdDelImage_Click()
- FullPathFileName = ""
- Image1.Picture = LoadPicture(App.Path & "\Images\NoImage.gif")
- End Sub
- Private Sub cmdExit_Click()
- Unload Me
- End Sub
- Private Sub Form_Unload(Cancel As Integer)
- Set frmLineNotify = Nothing
- End
- End Sub
คัดลอกไปที่คลิปบอร์ด
ดาวน์โหลดโค้ดต้นฉบับ VB6 (SP6) ได้ที่นี่ ...
|
ขออภัย! โพสต์นี้มีไฟล์แนบหรือรูปภาพที่ไม่ได้รับอนุญาตให้คุณเข้าถึง
คุณจำเป็นต้อง ลงชื่อเข้าใช้ เพื่อดาวน์โหลดหรือดูไฟล์แนบนี้ คุณยังไม่มีบัญชีใช่ไหม? ลงทะเบียน
x
|