|
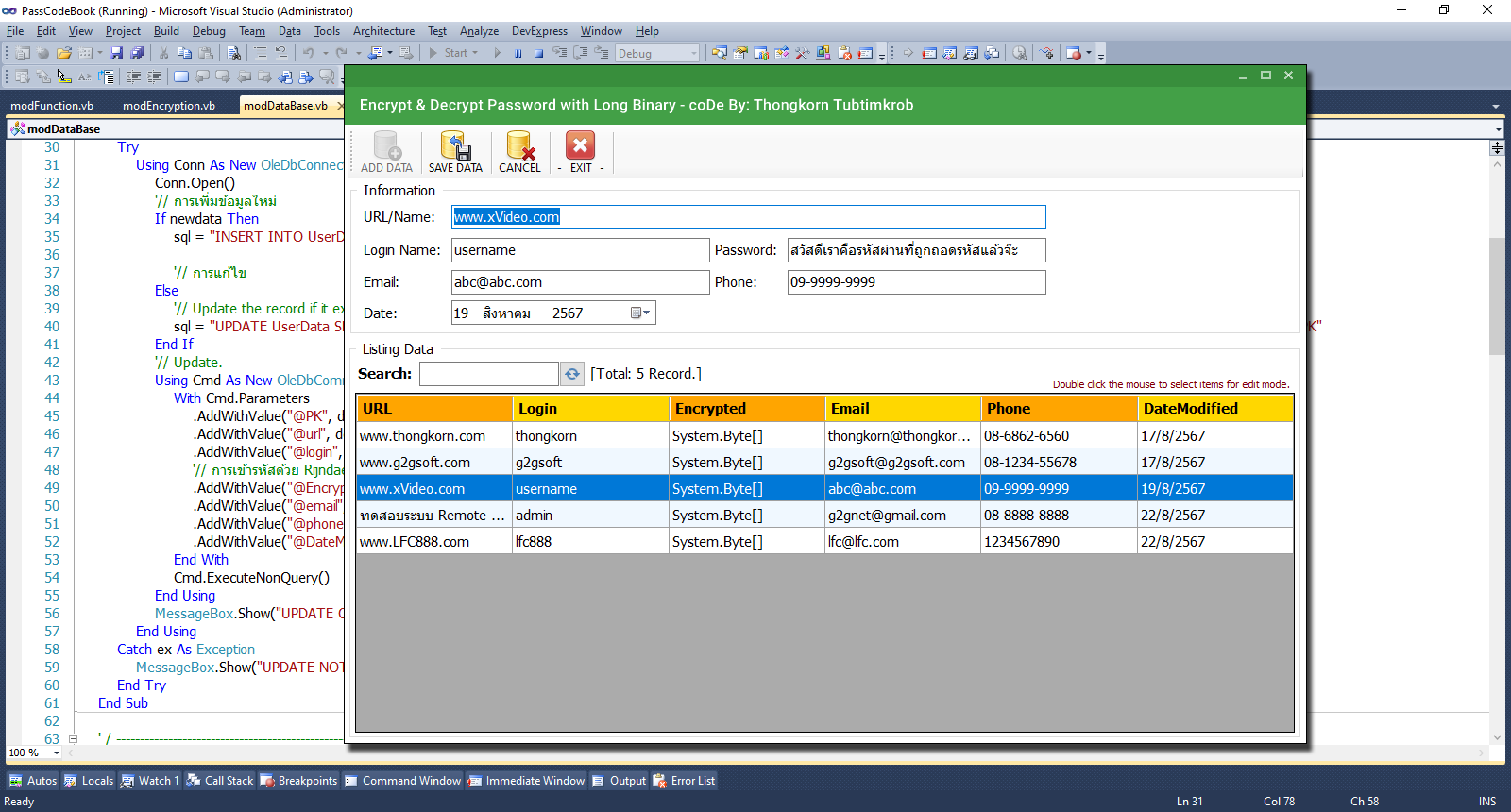
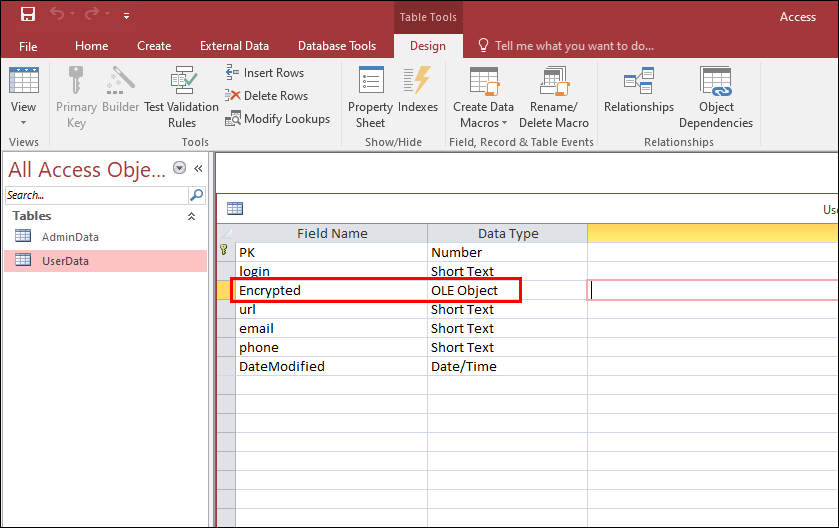
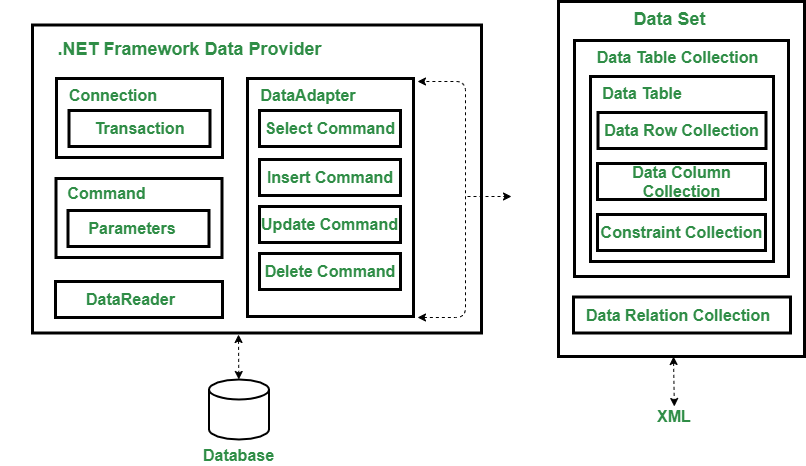
โค้ดโปรแกรมการเก็บข้อมูลรหัสผ่านด้วยการเข้ารหัสแบบ Long Binary หรือชนิดข้อมูลแบบ OLE Object สำหรับ MS Access โดยจะใช้การเข้ารหัสแบบ Rijndael (AES) Algorithm ... สำหรับการจัดการกับฐานข้อมูลเรียกว่า Parameterized Query หรือการใช้คำสั่งที่มีการส่งผ่านค่าไปยังตัวแปรพารามิเตอร์ (Parameterization) โดยตรงในการเขียน SQL Query ซึ่งเป็นวิธีที่ช่วยป้องกันการโจมตีแบบ SQL Injection ได้ดี ... โค้ดชุดนี้แอดมินเพิ่มความสวยงามด้วยการใช้ MaterialSkin เข้ามาช่วย ...
มาดูโค้ดฉบับเต็มจากฟอร์มหลัก (frmPassCodeBook.vb)
- Imports System.Data.OleDb
- Imports MaterialSkin
- Public Class frmPassCodeBook
- '// Add new or Edit data.
- Dim blnNewData As Boolean = False '// Edit mode.
- Dim PK As Long '// เก็บค่า Primary Key ทั้งการเพิ่มและแก้ไขข้อมูล
- ' / ------------------------------------------------------------------------------------------------
- ' / S T A R T ... H E R E
- ' / ------------------------------------------------------------------------------------------------
- Private Sub frmPassCodeBook_Load(sender As System.Object, e As System.EventArgs) Handles MyBase.Load
- '// Initialize MaterialSkin .Net Framework 4.0
- Dim SkinManager As MaterialSkinManager = MaterialSkinManager.Instance
- SkinManager.AddFormToManage(Me)
- SkinManager.Theme = MaterialSkinManager.Themes.LIGHT
- '//SkinManager.ColorScheme = New MaterialSkin.ColorScheme(Primary.Amber500, Primary.BlueGrey900, Primary.BlueGrey500, Accent.LightBlue200, TextShade.WHITE)
- SkinManager.ColorScheme = New MaterialSkin.ColorScheme(Primary.Green600, Primary.Green700, Primary.Green200, Accent.Red100, TextShade.WHITE)
- '// ตั้งค่าขนาดของฟอร์มแบบ Run Time
- Me.Size = New Size(1020, 720)
- '// คำนวณตำแหน่งที่กึ่งกลางของหน้าจอ
- Me.Location = New Point((Screen.PrimaryScreen.WorkingArea.Width - Me.Width) \ 2,
- (Screen.PrimaryScreen.WorkingArea.Height - Me.Height) \ 2)
- '// โหลดข้อมูลลงตารางกริด
- dgvData.DataSource = RetrieveData()
- Call SetupDataGridView(dgvData)
- lblRecordCount.Text = "[Total: " & dgvData.Rows.Count & " Record.]"
- '// เริ่มโหมดว่างเปล่าเพื่อทำรายการใหม่ หรือเลือกรายการขึ้นมาแก้ไข
- Call NewMode()
- End Sub
- ' / ------------------------------------------------------------------------------------------------
- ' / Add New Mode
- ' / ------------------------------------------------------------------------------------------------
- Private Sub NewMode()
- '// Clear all TextBox.
- For Each c In GroupBox1.Controls
- If TypeOf c Is TextBox Then
- DirectCast(c, TextBox).Clear()
- DirectCast(c, TextBox).Enabled = False
- End If
- If TypeOf c Is DateTimePicker Then DirectCast(c, DateTimePicker).Enabled = False
- Next
- itemAdd.Enabled = True
- itemSave.Enabled = False
- itemDelete.Enabled = True
- itemDelete.Text = "DELETE DATA"
- itemExit.Enabled = True
- End Sub
- ' / ------------------------------------------------------------------------------------------------
- ' / Edit Data Mode
- ' / ------------------------------------------------------------------------------------------------
- Private Sub EditMode()
- '// Clear all TextBox
- For Each c In GroupBox1.Controls
- If TypeOf c Is TextBox Then
- DirectCast(c, TextBox).Enabled = True
- End If
- If TypeOf c Is DateTimePicker Then DirectCast(c, DateTimePicker).Enabled = True
- Next
- itemAdd.Enabled = False
- itemSave.Enabled = True
- itemDelete.Enabled = True
- itemDelete.Text = "CANCEL"
- End Sub
- ' / ------------------------------------------------------------------------------------------------
- ' / Add new data.
- ' / ------------------------------------------------------------------------------------------------
- Private Sub itemAdd_Click(sender As System.Object, e As System.EventArgs) Handles itemAdd.Click
- blnNewData = True
- Call EditMode()
- txtUrl.Focus()
- End Sub
- ' / ------------------------------------------------------------------------------------------------
- ' / Save Data.
- ' / ------------------------------------------------------------------------------------------------
- Private Sub itemSave_Click(sender As System.Object, e As System.EventArgs) Handles itemSave.Click
- If txtUrl.Text = "" Or txtUrl.Text.Trim.Length = 0 Then
- MessageBox.Show("Please enter Url or Name.", "Report Status", MessageBoxButtons.OK, MessageBoxIcon.Warning)
- txtUrl.Focus()
- Return
- End If
- If txtLogin.Text = "" Or txtLogin.Text.Trim.Length = 0 Then
- MessageBox.Show("Please enter Login Name.", "Report Status", MessageBoxButtons.OK, MessageBoxIcon.Warning)
- txtLogin.Focus()
- Return
- End If
- If txtPassword.Text = "" Or txtPassword.Text.Trim.Length = 0 Then
- MessageBox.Show("Please enter Password.", "Report Status", MessageBoxButtons.OK, MessageBoxIcon.Warning)
- txtPassword.Focus()
- Return
- End If
- '// Details Class in modDataBase.vb
- '// กำหนดค่าให้กับตัวแปร เพื่อส่งออกไปทำการบันทึกข้อมูล
- Dim result As New Details
- With result
- .PK = PK
- .Login = txtLogin.Text
- .Encrypted = txtPassword.Text
- .Url = txtUrl.Text
- .Email = txtEmail.Text
- .Phone = txtPhone.Text
- .DateModified = dtpDateModified.Value
- End With
- '// blnNewData = True คือการเพิ่มข้อมูล
- If blnNewData Then
- '// หาค่า Primary Key จาก SetupNewPK อยู่ใน modDataBase.vb
- result.PK = SetupNewPK("SELECT MAX(UserData.PK) AS MaxPK FROM UserData")
- '// ส่งค่า blnNewData = True บอกว่าเป็นการเพิ่มข้อมูลใหม่ และค่าของ Control บนฟอร์มไปด้วย
- Call SaveData(blnNewData, result)
- Call NewMode()
- '// การแก้ไขข้อมูล
- Else
- 'blnNewData = False
- '// ส่งค่า blnNewData = False บอกว่าเป็นการเพิ่มข้อมูลใหม่ และส่งค่าของ Control บนฟอร์มไปด้วย
- Call SaveData(blnNewData, result)
- Call NewMode()
- End If
- '// Refresh data.
- Call btnRefresh_Click(sender, e)
- End Sub
- ' / ------------------------------------------------------------------------------------------------
- ' / DELETE DATA
- ' / ------------------------------------------------------------------------------------------------
- Private Sub itemDelete_Click(sender As System.Object, e As System.EventArgs) Handles itemDelete.Click
- If itemDelete.Text = "DELETE DATA" Then
- '// เช็คก่อนว่ามีรายแถวอยู่หรือไม่ หรือมีการคลิ๊กเลือกแถวรายการหรือเปล่า
- If dgvData.RowCount = 0 Or dgvData.CurrentRow Is Nothing Then Exit Sub
- Dim Url As String = dgvData.Item(1, dgvData.CurrentRow.Index).Value
- '// ถามยืนยันการลบข้อมูล
- Dim Result As Byte = MessageBox.Show("Are you sure you want to delete the data?" & vbCrLf & "URL/Name: " & Url, "Confirm Deletion", MessageBoxButtons.YesNo, MessageBoxIcon.Question, MessageBoxDefaultButton.Button2)
- If Result = DialogResult.Yes Then
- '// Receive Primary Key value to confirm the deletion.
- PK = dgvData.Item(0, dgvData.CurrentRow.Index).Value
- '// ลบข้อมูล (modDataBase.vb)
- Call DeleteData(PK)
- '// ทำการแสดงผลรายการใหม่
- Call btnRefresh_Click(sender, e)
- Call NewMode()
- End If
- ElseIf itemDelete.Text = "CANCEL" Then
- '// เริ่มโหมดว่างเปล่าเพื่อทำรายการใหม่ หรือเลือกรายการขึ้นมาแก้ไข
- Call NewMode()
- End If
- End Sub
- ' / ------------------------------------------------------------------------------------------------
- ' / แสดงผลข้อมูลทั้งหมด
- ' / ------------------------------------------------------------------------------------------------
- Private Sub btnRefresh_Click(sender As System.Object, e As System.EventArgs) Handles btnRefresh.Click
- dgvData.DataSource = RetrieveData()
- lblRecordCount.Text = "[Total: " & dgvData.Rows.Count & " Record.]"
- End Sub
- ' / ------------------------------------------------------------------------------------------------
- ' / การค้นหาข้อมูล
- ' / ------------------------------------------------------------------------------------------------
- Private Sub txtSearch_KeyPress(sender As Object, e As System.Windows.Forms.KeyPressEventArgs) Handles txtSearch.KeyPress
- '// Undesirable characters for the database ex. ', * or %
- txtSearch.Text = txtSearch.Text.Replace("'", "").Replace("*", "").Replace("%", "")
- If Trim(txtSearch.Text) = "" Or Len(Trim(txtSearch.Text)) = 0 Then Exit Sub
- '// RetrieveData(True) It means searching for information.
- If e.KeyChar = Chr(13) Then '// Press Enter
- '// No beep.
- e.Handled = True
- '//
- dgvData.DataSource = RetrieveData(True, txtSearch.Text)
- lblRecordCount.Text = "[Total: " & dgvData.Rows.Count & " Record.]"
- txtSearch.Clear()
- End If
- End Sub
- ' / ------------------------------------------------------------------------------------------------
- ' / ดับเบิ้ลคิ๊กเมาส์ในตารางกริด จากนั้นค้นหา Primary Key เพื่อนำข้อมูลไปแสดงผล
- ' / ------------------------------------------------------------------------------------------------
- Private Sub dgvData_CellDoubleClick(sender As Object, e As System.Windows.Forms.DataGridViewCellEventArgs) Handles dgvData.CellDoubleClick
- If e.RowIndex >= 0 Then
- '// เก็บค่านี้เอาไว้เพื่อใช้ในการอ้างอิงตอนที่ทำการอัพเดตข้อมูล
- PK = CInt(dgvData.Rows(e.RowIndex).Cells("PK").Value)
- blnNewData = False '// กำหนดให้เป็นการแก้ไขข้อมูล
- '// ส่งค่า PK เพื่อเป็นคีย์ไปทำการค้นข้อมูล แล้วคืนข้อมูลกลับมาในรูปแบบ Class
- '// Class Details อยู่ใน modDataBase.vb
- Dim result As Details = RetrieveDetails(PK)
- txtLogin.Text = result.Login
- txtPassword.Text = result.Encrypted
- txtUrl.Text = result.Url
- txtEmail.Text = result.Email
- txtPhone.Text = result.Phone
- dtpDateModified.Text = Format(result.DateModified, "dd/MM/yyyy")
- '// โหมดในการแก้ไข
- Call EditMode()
- txtUrl.Focus()
- End If
- End Sub
- '// Function to handle the KeyPress event for TextBox controls.
- Private Sub TextBox_KeyPress(sender As Object, e As KeyPressEventArgs) Handles txtLogin.KeyPress, txtPassword.KeyPress, txtUrl.KeyPress, txtEmail.KeyPress, txtPhone.KeyPress
- If e.KeyChar = ChrW(Keys.Enter) Then
- e.Handled = True '// Prevent the Enter key from adding a new line in the TextBox.
- SendKeys.Send("{TAB}") '// Send the TAB key to move to the next control.
- End If
- End Sub
- Private Sub dtpDateModified_KeyDown(sender As Object, e As System.Windows.Forms.KeyEventArgs) Handles dtpDateModified.KeyDown
- If e.KeyCode = Keys.Enter Then
- e.Handled = True
- SendKeys.Send("{TAB}")
- End If
- End Sub
- Private Sub frmPassCodeBook_FormClosed(sender As Object, e As System.Windows.Forms.FormClosedEventArgs) Handles Me.FormClosed
- Me.Dispose()
- GC.SuppressFinalize(Me)
- End
- End Sub
- Private Sub itemExit_Click(sender As System.Object, e As System.EventArgs) Handles itemExit.Click
- Me.Close()
- End Sub
- End Class
คัดลอกไปที่คลิปบอร์ด
โค้ดในส่วนของการจัดการฐานข้อมูล (modDataBase.vb)
- Imports System.Data.OleDb
- Module modDataBase
- Dim ConnString As String = "Provider=Microsoft.ACE.OLEDB.12.0;Data Source=" & MyPath(Application.StartupPath) & "\PassCodeBook.accdb;"
- ' / ------------------------------------------------------------------------------------------------
- '// การใช้ Key และ IV
- '// Key ควรมีขนาด 16, 24 หรือ 32 bytes เพื่อความปลอดภัย
- '// IV (Initialization Vector) ควรมีขนาด 16 bytes สำหรับการเข้ารหัส AES
- Dim key As String = "1234567890123456" '// Key 16 bytes
- Dim iv As String = "6543210987654321" '// IV 16 bytes
- ' / ------------------------------------------------------------------------------------------------
- Public Class Details
- '// Auto Implemented Properties for Data Fields.
- Public Property PK As Integer
- Public Property Login As String
- Public Property Encrypted As String
- Public Property Url As String
- Public Property Email As String
- Public Property Phone As String
- Public Property DateModified As Date
- End Class
- ' / ------------------------------------------------------------------------------------------------
- '// Save data to MS Access database.
- ' / ------------------------------------------------------------------------------------------------
- Sub SaveData(newdata As Boolean, details As Details)
- Dim sql As String
- Try
- Using Conn As New OleDbConnection(ConnString)
- Conn.Open()
- '// การเพิ่มข้อมูลใหม่
- If newdata Then
- sql = "INSERT INTO UserData (PK, url, Login, Encrypted, email, phone, DateModified) VALUES (@PK, @Url, @Email, @Phone, @Login, @Encrypted, @DateModified)"
- '// การแก้ไข
- Else
- '// Update the record if it exists.
- sql = "UPDATE UserData SET PK = @PK, url = @Url, Login = @Login, Encrypted = @Encrypted, email = @Email, Phone = @Phone, DateModified = @DateModified WHERE PK = @PK"
- End If
- '// Update.
- Using Cmd As New OleDbCommand(sql, Conn)
- With Cmd.Parameters
- .AddWithValue("@PK", details.PK)
- .AddWithValue("@url", details.Url)
- .AddWithValue("@login", details.Login)
- '// การเข้ารหัสด้วย Rijndael (AES) (modEncryption.vb)
- .AddWithValue("@Encrypted", EncryptPassword(details.Encrypted, key, iv))
- .AddWithValue("@email", details.Email)
- .AddWithValue("@phone", details.Phone)
- .AddWithValue("@DateModified", Format(details.DateModified, "dd/MM/yyyy"))
- End With
- Cmd.ExecuteNonQuery()
- End Using
- MessageBox.Show("UPDATE COMPLETE.", "Report Status", MessageBoxButtons.OK, MessageBoxIcon.Information)
- End Using
- Catch ex As Exception
- MessageBox.Show("UPDATE NOT SUCCESSFUL!", "Report Status", MessageBoxButtons.OK, MessageBoxIcon.Warning)
- End Try
- End Sub
- ' / ------------------------------------------------------------------------------------------------
- '// Retrieve data from MS Access database and display in DataGridView.
- '// blnSearch = True คือการค้นหาข้อมูล โดยรับค่า SearchValue เป็นคีย์เวิร์ดใช้ในการค้นหา
- '// blnSearch = False ก็จะแสดงผลข้อมูลออกมาทั้งหมด
- '// ทั้ง 2 ค่าเป็น Optional คือหากไม่ระบุการส่งค่ามา ก็ให้มีค่าเป็น False หรือแสดงผลข้อมูลออกมาทั้งหมด
- ' / ------------------------------------------------------------------------------------------------
- Function RetrieveData(Optional ByVal blnSearch As Boolean = False, Optional ByVal SearchValue As String = "") As DataTable
- Using Conn As New OleDbConnection(ConnString)
- Conn.Open()
- Dim sql As String = "SELECT PK, url, login, Encrypted, email, phone, DateModified FROM UserData"
- If blnSearch Then
- sql &= " WHERE url LIKE @SearchValue OR email LIKE @SearchValue OR phone LIKE @SearchValue OR login LIKE @SearchValue"
- End If
- sql &= " ORDER BY PK"
- '//
- Using cmd As New OleDbCommand(sql, Conn)
- If blnSearch Then cmd.Parameters.AddWithValue("@SearchValue", "%" & SearchValue & "%")
- '//
- Using reader As OleDbDataReader = cmd.ExecuteReader()
- Dim dt As New DataTable()
- With dt.Columns
- .Add("PK", GetType(Integer))
- .Add("URL", GetType(String))
- .Add("Login", GetType(String))
- .Add("Encrypted", GetType(String))
- .Add("Email", GetType(String))
- .Add("Phone", GetType(String))
- .Add("DateModified", GetType(Date))
- End With
- While reader.Read()
- '// มีการเข้ารหัสของรหัสผ่านเอาไว้ตอนแสดงผลในตารางกริด
- Dim EncryptedData As Byte() = DirectCast(reader("Encrypted"), Byte())
- '// เพิ่มรายการแถวข้อมูลเข้าไปใน DataTable
- dt.Rows.Add(reader("PK"), reader("url"), reader("login"), EncryptedData, reader("email"), reader("phone"), reader("DateModified"))
- End While
- Return dt
- End Using
- End Using
- End Using
- End Function
- ' / ------------------------------------------------------------------------------------------------
- ' / คืนค่าข้อมูลจากตารางกริดไปแสดงผลลงใน Control
- ' / ------------------------------------------------------------------------------------------------
- Public Function RetrieveDetails(PK As Integer) As Details
- '// Class Details เพื่อคืนค่ากลับไปแสดงผลในฟอร์ม
- Dim result As New Details()
- Try
- Using Conn As New OleDbConnection(ConnString)
- Conn.Open()
- Using cmd As New OleDbCommand("SELECT url, login, Encrypted, email, phone, DateModified FROM UserData WHERE PK = @PK", Conn)
- cmd.Parameters.AddWithValue("@PK", PK)
- Using reader As OleDbDataReader = cmd.ExecuteReader()
- If reader.Read() Then
- result.Url = reader("url").ToString()
- result.Login = reader("login").ToString()
- '// ถอดรหัสออกมาเพื่อให้เห็น Password แบบ Plain Text.
- result.Encrypted = DecryptPassword(DirectCast(reader("Encrypted"), Byte()), key, iv)
- result.Email = reader("email").ToString()
- result.Phone = reader("phone").ToString()
- result.DateModified = reader("DateModified").ToString()
- Return (result)
- End If
- End Using
- End Using
- End Using
- Catch ex As Exception
- MessageBox.Show("Error: " & ex.Message, "Report Status", MessageBoxButtons.OK, MessageBoxIcon.Warning)
- End Try
- Return result '// Return the initialized structure if no data is found.
- End Function
- ' / ------------------------------------------------------------------------------------------------
- ' / DELETE DATA
- ' / ------------------------------------------------------------------------------------------------
- Public Sub DeleteData(PK As Integer)
- Try
- Using Conn As New OleDbConnection(ConnString)
- Conn.Open()
- Dim sql As String = "DELETE FROM UserData WHERE PK = @PK"
- Using cmd As New OleDbCommand(sql, Conn)
- cmd.Parameters.AddWithValue("@PK", PK)
- Try
- cmd.ExecuteNonQuery()
- MessageBox.Show("DELETE COMPLETE.", "Report Status", MessageBoxButtons.OK, MessageBoxIcon.Information)
- Catch ex As Exception
- MessageBox.Show("Error: " & ex.Message)
- End Try
- End Using
- End Using
- Catch ex As Exception
- MessageBox.Show("Error: " & ex.Message, "Report Status", MessageBoxButtons.OK, MessageBoxIcon.Warning)
- End Try
- End Sub
- ' / ------------------------------------------------------------------------------------------------
- ' / Function to find and create the new Primary Key not to duplicate.
- ' / ------------------------------------------------------------------------------------------------
- Function SetupNewPK(ByVal sql As String) As Integer
- Using Conn As New OleDbConnection(ConnString)
- Conn.Open()
- Using cmd As New OleDbCommand(sql, Conn)
- '/ Check if the information is available. And return it back
- If IsDBNull(cmd.ExecuteScalar) Then
- '// Start at 1
- SetupNewPK = 1
- Else
- SetupNewPK = cmd.ExecuteScalar + 1
- End If
- End Using
- End Using
- End Function
- End Module
คัดลอกไปที่คลิปบอร์ด
โค้ดในส่วนของการเข้ารหัสและถอดรหัส (modEncryption.vb)
- Imports System.Security.Cryptography
- Imports System.Text
- Imports System.IO
- Module modEncryption
- '// Rijndael (AES) ในการเข้ารหัสข้อมูล (Encryption) โดยการใช้ Key และ IV (Initialization Vector).
- Public Function EncryptPassword(password As String, key As String, iv As String) As Byte()
- Dim aes As New RijndaelManaged()
- aes.Key = Encoding.UTF8.GetBytes(key)
- aes.IV = Encoding.UTF8.GetBytes(iv)
- aes.Padding = PaddingMode.PKCS7
- aes.Mode = CipherMode.CBC
- Dim encryptor As ICryptoTransform = aes.CreateEncryptor(aes.Key, aes.IV)
- Using ms As New MemoryStream()
- Using cs As New CryptoStream(ms, encryptor, CryptoStreamMode.Write)
- Using sw As New StreamWriter(cs)
- sw.Write(password)
- End Using
- End Using
- Return ms.ToArray()
- End Using
- End Function
- '// Rijndael (AES) ในการถอดรหัสข้อมูล (Decryption)
- Public Function DecryptPassword(encryptedPassword As Byte(), key As String, iv As String) As String
- Dim aes As New RijndaelManaged()
- aes.Key = Encoding.UTF8.GetBytes(key)
- aes.IV = Encoding.UTF8.GetBytes(iv)
- aes.Padding = PaddingMode.PKCS7
- aes.Mode = CipherMode.CBC
- Dim decryptor As ICryptoTransform = aes.CreateDecryptor(aes.Key, aes.IV)
- Using ms As New MemoryStream(encryptedPassword)
- Using cs As New CryptoStream(ms, decryptor, CryptoStreamMode.Read)
- Using sr As New StreamReader(cs)
- Return sr.ReadToEnd()
- End Using
- End Using
- End Using
- End Function
- End Module
คัดลอกไปที่คลิปบอร์ด
โค้ดในส่วนของฟังค์ชั่น (modFunction.vb)
- Module modFunction
- Function MyPath(ByVal AppPath As String) As String
- '/ Return Value
- MyPath = AppPath.ToLower.Replace("\bin\debug", "").Replace("\bin\release", "").Replace("\bin\x86\debug", "").Replace("\bin\x86\release", "")
- '// If not found folder then put the \ (BackSlash) at the end.
- If Microsoft.VisualBasic.Right(MyPath, 1) <> Chr(92) Then MyPath = MyPath & Chr(92)
- End Function
- ' / --------------------------------------------------------------------------------
- '// Initialize DataGridView @Run Time
- Public Sub SetupDataGridView(DGV As DataGridView)
- With DGV
- .RowHeadersVisible = False
- .AllowUserToAddRows = False
- .AllowUserToDeleteRows = False
- .AllowUserToResizeRows = False
- .MultiSelect = False
- .SelectionMode = DataGridViewSelectionMode.FullRowSelect
- .ReadOnly = True
- .Font = New Font("Tahoma", 11, FontStyle.Regular)
- .RowTemplate.Height = 28
- .RowTemplate.Resizable = DataGridViewTriState.False
- .Columns(0).Visible = False '// Primary Key
- '// Autosize Column
- .AutoSizeColumnsMode = DataGridViewAutoSizeColumnsMode.Fill
- '// Even-Odd Color
- .AlternatingRowsDefaultCellStyle.BackColor = Color.AliceBlue
- '// COLUMN HEADER
- '// Adjust Header Styles
- With .ColumnHeadersDefaultCellStyle
- .BackColor = Color.Navy
- .ForeColor = Color.Black ' Color.White
- .Font = New Font("Tahoma", 11, FontStyle.Bold)
- End With
- '// Before you can adjust the height of the row.
- .ColumnHeadersHeightSizeMode = DataGridViewColumnHeadersHeightSizeMode.DisableResizing
- .ColumnHeadersHeight = 30
- '// Accept color background changes.
- .EnableHeadersVisualStyles = False
- For iCol = 0 To DGV.Columns.Count - 1
- '// Calculates odd and even numbers. If any integer divide by 2, then answer 1 is odd number.
- If iCol Mod 2 = 0 Then
- DGV.Columns(iCol).HeaderCell.Style.BackColor = Color.Gold
- '// Integer divide by 2, then answer 1 is Even number.
- Else
- DGV.Columns(iCol).HeaderCell.Style.BackColor = Color.Orange
- End If
- Next
- End With
- End Sub
- End Module
คัดลอกไปที่คลิปบอร์ด
ดาวน์โหลดโค้ดฉบับเต็ม VB.NET (2010) ได้ที่นี่ ...
|
ขออภัย! โพสต์นี้มีไฟล์แนบหรือรูปภาพที่ไม่ได้รับอนุญาตให้คุณเข้าถึง
คุณจำเป็นต้อง ลงชื่อเข้าใช้ เพื่อดาวน์โหลดหรือดูไฟล์แนบนี้ คุณยังไม่มีบัญชีใช่ไหม? ลงทะเบียน
x
|