|
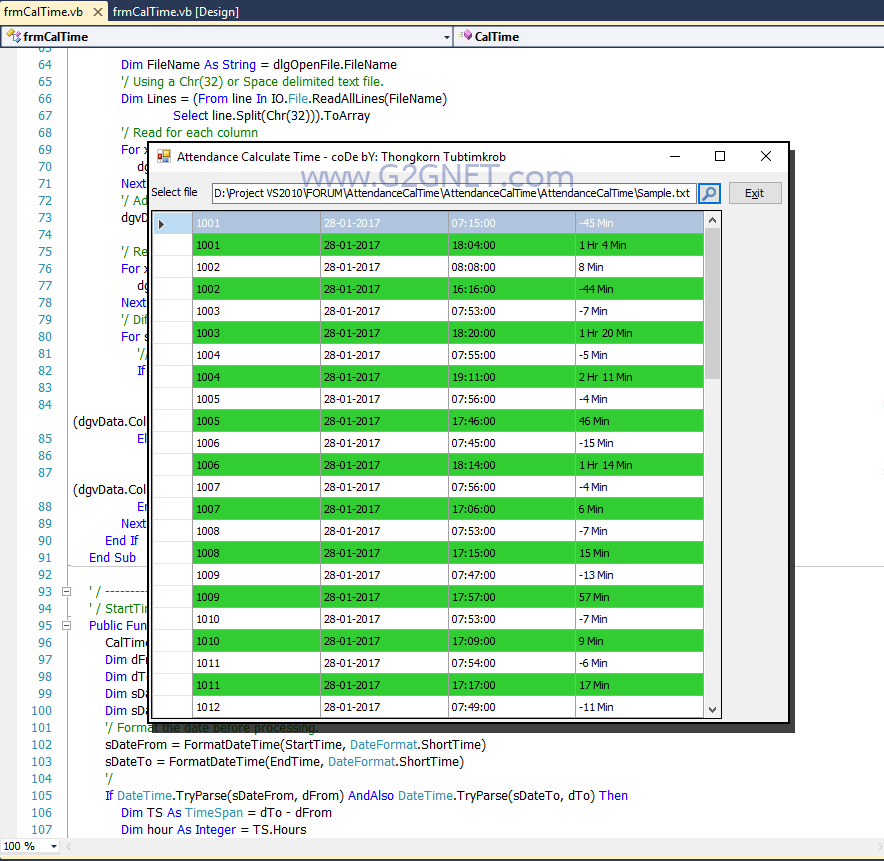
แอดมินเคยเขียนโค้ดแจกให้ไปแล้วทั้ง VB6 และ VBA โดยสามารถตามกลับไปอ่านได้จาก [VB6] การคำนวณหาเวลาการเข้าออกของพนักงาน (Time Attendance) ในระดับนาที และ [VBA] การคำนวณหาเวลาการเข้ามาทำงานของพนักงาน (Time Attendance) ... สำหรับตอนนี้จะเป็น VB.NET กันบ้างครับ
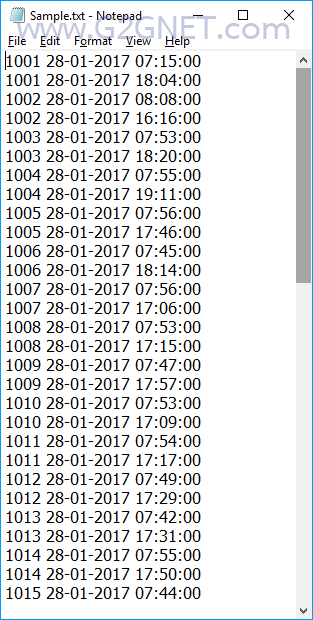
ข้อมูลที่ได้รับมาจากเครื่องสแกนลายนิ้วมือ (Finger Scan) ซึ่งชุดข้อมูลจะถูกแยกออกจากกันด้วย Space หรือ CHR(32)
มาดูโค้ดกันเถอะ ...
- ' / ------------------------------------------------------------------
- ' / Developer : Mr.Surapon Yodsanga (Thongkorn Tubtimkrob)
- ' / eMail : thongkorn@hotmail.com
- ' / URL: http://www.g2gnet.com (Khon Kaen - Thailand)
- ' / Facebook: https://www.facebook.com/g2gnet (For Thailand)
- ' / Facebook: https://www.facebook.com/commonindy (Worldwide)
- ' / Purpose: Time attendance & calculate difference time.
- ' / Microsoft Visual Basic .NET (2010)
- ' /
- ' / This is open source code under @CopyLeft by Thongkorn Tubtimkrob.
- ' / You can modify and/or distribute without to inform the developer.
- ' / ------------------------------------------------------------------
- Public Class frmCalTime
- ' / ------------------------------------------------------------------
- ' / Get my project path
- ' / AppPath = C:\My Project\bin\debug
- ' / Replace "\bin\debug" with ""
- ' / Return : C:\My Project\
- Function MyPath(ByVal AppPath As String) As String
- '/ MessageBox.Show(AppPath);
- AppPath = AppPath.ToLower()
- '/ Return Value
- MyPath = AppPath.Replace("\bin\debug", "").Replace("\bin\release", "").Replace("\bin\x86\debug", "")
- '// If not found folder then put the \ (BackSlash) at the end.
- If Microsoft.VisualBasic.Right(MyPath, 1) <> "" Then MyPath = MyPath & ""
- End Function
- ' / ------------------------------------------------------------------
- ' / Browse text file and calculate difference time.
- Private Sub btnBrowse_Click(sender As System.Object, e As System.EventArgs) Handles btnBrowse.Click
- '/ Declaration Open File Dialog @Run Time
- Dim dlgOpenFile As OpenFileDialog = New OpenFileDialog()
- ' / Open File Dialog
- With dlgOpenFile
- .InitialDirectory = MyPath(Application.StartupPath)
- .Title = "Select text file."
- .Filter = "Text Files (*.txt)|*.txt"
- .FilterIndex = 1
- .RestoreDirectory = True
- End With
- ' Choose OK button after Browse ...
- If dlgOpenFile.ShowDialog() = DialogResult.OK Then
- txtFileName.Text = dlgOpenFile.FileName
- ' / Initialize DataGridView
- With dgvData
- .ColumnHeadersVisible = False
- .AlternatingRowsDefaultCellStyle.BackColor = Color.LimeGreen
- .DefaultCellStyle.SelectionBackColor = Color.LightSteelBlue
- .SelectionMode = DataGridViewSelectionMode.FullRowSelect
- .RowTemplate.Height = 22
- .ReadOnly = True
- .MultiSelect = False
- .Columns.Clear()
- '// Autosize Column
- .AutoSizeColumnsMode = DataGridViewAutoSizeColumnsMode.Fill
- .AutoResizeColumns()
- End With
- Dim FileName As String = dlgOpenFile.FileName
- '/ Using a Chr(32) or Space delimited text file.
- Dim Lines = (From line In IO.File.ReadAllLines(FileName)
- Select line.Split(Chr(32))).ToArray
- '/ Read for each column
- For x As Integer = 0 To Lines(0).GetUpperBound(0)
- dgvData.Columns.Add(Lines(0)(x), Lines(0)(x))
- Next
- '/ Add new column for calculate time.
- dgvData.Columns.Add("CalDiff", "Difference Time")
- '/ Read line by line for each rows
- For x As Integer = 0 To Lines.GetUpperBound(0)
- dgvData.Rows.Add(Lines(x))
- Next
- '/ Difference Time
- For sRow = 0 To dgvData.RowCount - 1
- '// Time in
- If (sRow Mod 2) = 0 Then
- '// Compare with Time IN = 08:00 AM
- dgvData.Rows(sRow).Cells(dgvData.Columns.Count - 1).Value = CalTime("08:00", dgvData.Rows(sRow).Cells(dgvData.Columns.Count - 2).Value)
- Else
- '// Compare with Time OUT = 17:00 PM
- dgvData.Rows(sRow).Cells(dgvData.Columns.Count - 1).Value = CalTime("17:00", dgvData.Rows(sRow).Cells(dgvData.Columns.Count - 2).Value)
- End If
- Next
- End If
- End Sub
- ' / ------------------------------------------------------------------
- ' / StartTime is IN/OUT reference time, EndTime is actual employee time.
- Public Function CalTime(StartTime As DateTime, ByVal EndTime As DateTime) As String
- CalTime = ""
- Dim dFrom As DateTime
- Dim dTo As DateTime
- Dim sDateFrom As String
- Dim sDateTo As String
- '/ Format the date before processing.
- sDateFrom = FormatDateTime(StartTime, DateFormat.ShortTime)
- sDateTo = FormatDateTime(EndTime, DateFormat.ShortTime)
- '/
- If DateTime.TryParse(sDateFrom, dFrom) AndAlso DateTime.TryParse(sDateTo, dTo) Then
- Dim TS As TimeSpan = dTo - dFrom
- Dim hour As Integer = TS.Hours
- Dim mins As Integer = TS.Minutes
- Dim secs As Integer = TS.Seconds
- Dim TimeDiff As String = "0"
- If hour <> 0 Then
- TimeDiff = (hour.ToString & " Hr ") + mins.ToString & " Min"
- ElseIf mins <> 0 Then
- TimeDiff = mins.ToString & " Min"
- End If
- '/ You can defined to format the problem.
- '/ Sample: Output 16 mins in format 00:16:00
- 'TimeDiff = ((hour.ToString("00") & ":") + mins.ToString("00") & ":") + secs.ToString("00")
- CalTime = TimeDiff
- End If
- End Function
- Private Sub btnExit_Click(sender As System.Object, e As System.EventArgs) Handles btnExit.Click
- Me.Close()
- End Sub
- Private Sub frmCalTime_FormClosed(sender As Object, e As System.Windows.Forms.FormClosedEventArgs) Handles Me.FormClosed
- Me.Dispose()
- Application.Exit()
- End Sub
- Private Sub txtFileName_KeyPress(sender As Object, e As System.Windows.Forms.KeyPressEventArgs) Handles txtFileName.KeyPress
- '// Lock any key press.
- e.Handled = True
- End Sub
- End Class
คัดลอกไปที่คลิปบอร์ด
ดาวน์โหลดโค้ดต้นฉบับ VB.NET (2010) ได้ที่นี่ ...
|
ขออภัย! โพสต์นี้มีไฟล์แนบหรือรูปภาพที่ไม่ได้รับอนุญาตให้คุณเข้าถึง
คุณจำเป็นต้อง ลงชื่อเข้าใช้ เพื่อดาวน์โหลดหรือดูไฟล์แนบนี้ คุณยังไม่มีบัญชีใช่ไหม? ลงทะเบียน
x
|